- Electronics
- Xilinx
Acceleration (Part 2)
Since the bottle-neck appeared to be the SPI bus, I decided to have a go at reducing the number of bytes needed to do various things by extending the instruction set.
The instruction set now looks like this:
- 0x0X - NOP
- 0x10 - Set colour
- 0x11 - Set address
- 0x12 - Add 8-bit value to address
- 0x13 - Add 16-bit value to address
- 0x20 - Plot single pixel in current colour
- 0x21 - Plot given pixel value
- 0x22 - Draw horizontal line from 1 to 256 pixels
- 0x23 - Draw horizontal line from 1 to 65536 pixels
- 0x24 - Draw horizontal line in 24-bit value
- 0x30 - Draw pixel map
- 0x31 - Draw up to 255 given pixel colours
- 0x4X - Draw horizontal line from 1 to 16 pixels (X + 1)
- 0x5X - Add X to current address (X + 1)
All other commands are currently ignored (NOP).
The 0x4X commands did appear to make it go much faster.
Interrupts
I had almost decided to remove the interrupt code from the PicoBlaze processor, but then I had an idea - use the interrupt to pause the CPU while the write buffer was full. This would allow me to remove the code that waited for the FIFO to be empty, and have the processor just wait until the FIFO had gone below full before resuming.
In order to prevent the FIFO from being written while the interrupt was being processed, I added the "almost-full" flag to the FIFO, and used that as a trigger for the IRQ.
This worked very well (although didn't overly speed things up).
SPI code
I'd written a program to convert a 2bpp bitmap into a series of hline instructions - however, it was not optimised for the instructions being used.
I gave it some intelligence as to how the SPI stream is generated, and recalculated it.
This had two effects:
- The PIC code went from around 0x3200 bytes to 0x2200 bytes (which is a fair saving).
- It was now significantly faster that it was beforehand. Not quite instantaneous, but good enough!
Hexadecimal display
I don't have the memory for a full font, but I can display 7-segment like digits, which would be useful for some of the less important information (such as the engine temperature, boost pressure and the like). Since I've got some spare space in the PicoBlaze, I put in a generic 7-segment display command.
- 0x14 - Display 7-segment display (bits 6 to 0 of next data byte are segments G to A respectively)
This uses the current colour, but if a segment is turned off, then it uses a darker version (only primary colours are really supported for this):
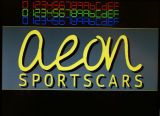
The really good thing about this is that the address is automatically adjusted to the next location, so in order to send a sequence of bytes, you only need to send the digits themselves.